Driver
The driver section allows developers to select drivers for various responsibilities of a TouchGFX AL.
Application Tick Source
The application tick source for an application defines how to drive an application forward. The developer has the following options:
- LTDC - If LTDC is selected as the interface in the "Display" group, the Application Tick Source can be "LTDC". This means that TouchGFX Generator will generate a driver function (LTDC interrupt handler) in
TouchGFXGeneratedHAL
class that drives the application forward by callingOSWrappers::signalVSync()
. - Custom and FMC - In this case, the developer is required to implement a handler that drives the application forward by calling
OSWrappers::signalVSync()
repeatedly.
Further reading
DMA2D Accelerator (Chrom-ART)
The developer has three options when it comes to DMA2D graphics acceleration:
- No - The application renders using only the CPU.
- Yes - The application uses the Chrom-ART chip when possible to move and blend pixels, freeing up CPU cycles. The driver is generated by TouchGFX Generator and does not require any action from the developer.
- Custom - TouchGFX Generator generates a generic TouchGFX DMA class that inherits from the DMA interface in the TouchGFX Engine with member functions that the developer must implement for a custom graphics accelerator.
To be able to select DMA2D Accelerator in TouchGFX Generator, it must be enabled in the Multimedia category in STM32CubeMX:
The DMA2D (Chrom-ART) driver generated by TouchGFX Generator supports two ways of receiving a TransferCompleteInterrupt:
- Uses the STM32Cube HAL driver where it registers a callback function to the dma2d handle
hdma2d.XferCpltCallback
. - Uses the
DMA2D_IRQHandler()
interrupt handler directly.
Switching between these two is done by enabling or disabling the DMA2D global interrupt in the NVIC Settings in STM32CubeMX for DMA2D IP:
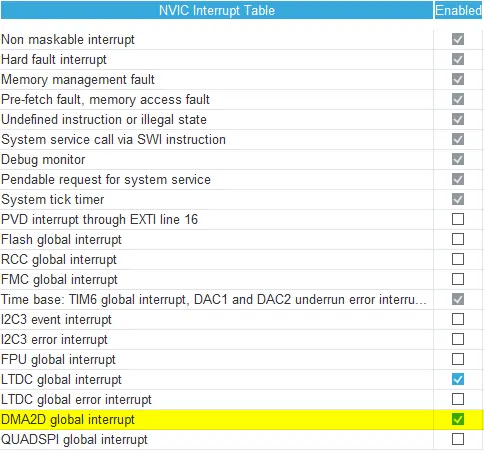
DMA2D global interrupt is enabled/disabled in the NVIC settings under System Core category in STM32CubeMX
Enabling the global interrupt generates code for option 1), disabling the global interrupt generates code for option 2).
Note
GPU2D Accelerator (NeoChrom)
GPU2D (NeoChrom) is a graphics accelerator capable of accelerating many of the drawing operations in TouchGFX, including texture mapping and Vector Rendering. It supports framebuffers in RGB565, RGB888, and ARGB8888 formats.
The developer has two options when it comes to GPU2D graphics acceleration:
- No - The application draws using only the CPU.
- Yes - The application uses the NeoChrom chip when possible to accelerate many drawing operations done by TouchGFX. The driver is generated by TouchGFX Generator and does not require any action from the developer.
To be able to select GPU2D Accelerator in TouchGFX Generator, it must be enabled in the Multimedia category in STM32CubeMX:
Note
When enabled, a new section (GPU2D Driver) will appear in the TouchGFX Generator:
The setting GPU2D Command List Size sets the size in bytes of the command list used by the GPU2D.
Note
Real-Time Operating System
Developers can use any RTOS with TouchGFX (even No OS). As described in Abstraction Layer Architecture the
TouchGFX Engine uses the OSWrappers
interface to synchronize its main event loop as well as framebuffer(s) access with the users choice
of RTOS. When developers choose an operating system in TouchGFX Generator, code will be generated to synchronize internally via primitives
for the OS of choice. The operating system still has to be configured through STM32CubeMX to determine stack size, among other things.
FreeRTOS (CMSIS OS V1 and V2) and ThreadX (Native Middleware or Azure RTOS Software Packs) can be configured directly from within STM32CubeMX and TouchGFX Generator provides the user with generated code for both task definitions and TouchGFX RTOS driver. TouchGFX Generator can generate CMSIS V1 and CMSIS V2 compliant RTOS drivers which work with any CMSIS compliant RTOS, a driver for ThreadX, and a driver for running bare metal without an operating system (No OS).
No OS
If it is desired to run TouchGFX with no operating system, the No OS option in TouchGFX Generator becomes available if no operating system is enabled in the Middleware and Software Packs category, or selected as an X-CUBE in STM32CubeMX.
Further reading
FreeRTOS
To be able to select CMSIS_RTOS_V1 or CMSIS_RTOS_V2 in TouchGFX Generator, FreeRTOS must be enabled in the Middleware and Software Packs category in STM32CubeMX:
Some TouchGFX Board Setups are not configured to use FreeRTOS Middleware by default, but ThreadX or NoOS (e.g. STM32U599). If you still want to use FreeRTOS with these TouchGFX Board Setups, you must download the X-CUBE-FreeRTOS pack in the Software Packs Components Selector of STM32CubeMX, and enable it.
Further reading
ThreadX
ThreadX can be enabled either by selecting a X-CUBE Software Pack or by enabling the native ThreadX Middleware from STM32CubeMX, if available for the selected MCU device.
The developer should find out if ThreadX is configured as an X-CUBE Software Pack or as native ThreadX Middleware for their specific MCU family.
Note
The following sections will show how to enable ThreadX as a Middleware or as an AZRTOS Software Pack for your application.
Enabling ThreadX from Middleware and Software Packs category
To be able to select ThreadX in TouchGFX Generator, ThreadX must be enabled in the Middleware and Software Packs category in STM32CubeMX.
Once enabled, the ThreadX option becomes available in TouchGFX Generator.
Further reading
Enabling ThreadX from X-CUBE Software Pack
To be able to select ThreadX in TouchGFX Generator the AZRTOS Software Pack must be enabled in the Software Packs Component Selector in STM32CubeMX.
In your project click the "Select Components" button or Alt + o
in the project menu in STM32CubeMX.
The following figure shows how AZRTOS Software Pack can be enabled for a project. Select RTOS ThreadX and tick the Core check box to enable ThreadX. Download and Install the package if not already done.
After having added ThreadX Software Pack to the project, the X-CUBE should be visible under the Middleware and Software Packs category on the left side of the project menu in STM32CubeMX.
Once ThreadX is enabled, it also becomes an available option in TouchGFX Generator, and after selecting it the Memory Pool Size and Memory Stack Size values will appear:
Those values should be large enough to contain threads ressources.
Further reading
Other CMSIS compliant OS
When developers require a different CMSIS compliant OS than what STM32CubeMX can offer (FreeRTOS and ThreadX)
they must perform RTOS configuration and task definition manually. TouchGFX Generator will generate an empty
OSWrappers
interface that the developer must implement. Generally, the following manual steps are required:
- Configure the RTOS
- Implement
OSWrappers
interface - Define a task to run TouchGFX (
MX_TouchGFX_Process
) - Start the scheduler
Call MX_TouchGFX_Process
to start the TouchGFX Engine Main Loop inside the task handler.
void MX_TouchGFX_Process(void);